Package panamagl.canvas
Class GLCanvasSwing
java.lang.Object
java.awt.Component
java.awt.Container
javax.swing.JComponent
javax.swing.JPanel
panamagl.canvas.GLCanvasSwing
- All Implemented Interfaces:
ImageObserver
,MenuContainer
,Serializable
,Accessible
,GLCanvas
This panel push to the screen an OpenGL image rendered offscreen by an
OffscreenRenderer
.
The panel mainly deals with
- OpenGL context initialization, after the panel is added to a parent, and before it is made visible.
- repaint and resize events that are propagated to the OpenGL application through a
GLEventListener
, which must be provided by the user of this panel throughsetGLEventListener(GLEventListener)
. - measuring performance, that is evaluating the time required to trigger offscreen image rendering and image painting onscreen.
Threading
The panel is also responsible for triggering OpenGL initialization and rendering in the appropriate threads, which may depend on the running operating system.Threading on macOS
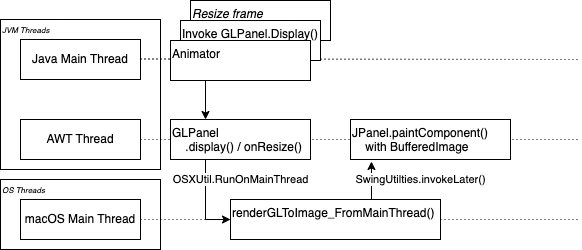
Debugging
Hint : to debug this class, invoke a program using it with flag -Dpanamagl.GLPanel- Author:
- Martin Pernollet
- See Also:
-
Nested Class Summary
Nested ClassesModifier and TypeClassDescriptionprotected class
TheGLCanvasSwing.ResizeHandler
will trigger rendering on the main macOS thread and then trigger repaint throughinvalid @link
{@link SwingUtilities.invokeLater()
Nested classes/interfaces inherited from class javax.swing.JPanel
JPanel.AccessibleJPanel
Nested classes/interfaces inherited from class javax.swing.JComponent
JComponent.AccessibleJComponent
Nested classes/interfaces inherited from class java.awt.Container
Container.AccessibleAWTContainer
Nested classes/interfaces inherited from class java.awt.Component
Component.AccessibleAWTComponent, Component.BaselineResizeBehavior, Component.BltBufferStrategy, Component.FlipBufferStrategy
Nested classes/interfaces inherited from interface panamagl.canvas.GLCanvas
GLCanvas.Flip
-
Field Summary
FieldsModifier and TypeFieldDescriptionprotected RenderCounter
protected boolean
protected boolean
protected GLCanvas.Flip
protected GLEventListener
protected OffscreenRenderer
protected BufferedImage
protected PerformanceOverlay_AWT
protected AtomicBoolean
Fields inherited from class javax.swing.JComponent
listenerList, TOOL_TIP_TEXT_KEY, ui, UNDEFINED_CONDITION, WHEN_ANCESTOR_OF_FOCUSED_COMPONENT, WHEN_FOCUSED, WHEN_IN_FOCUSED_WINDOW
Fields inherited from class java.awt.Component
accessibleContext, BOTTOM_ALIGNMENT, CENTER_ALIGNMENT, LEFT_ALIGNMENT, RIGHT_ALIGNMENT, TOP_ALIGNMENT
Fields inherited from interface java.awt.image.ImageObserver
ABORT, ALLBITS, ERROR, FRAMEBITS, HEIGHT, PROPERTIES, SOMEBITS, WIDTH
-
Constructor Summary
ConstructorsConstructorDescriptionGLCanvasSwing
(PanamaGLFactory factory) Initialize a panel able to render OpenGL through aGLEventListener
and relatedGL
interface. -
Method Summary
Modifier and TypeMethodDescriptionvoid
Called after the JPanel has been added to the Swing hierarchy but before it is made visible.void
display()
If the panel initialization has achieved, this triggers an offscreen rendering, maybe on a separated thread (macOS case), from which an asynchronous repaint will be triggered.Return the GL context.getFBO()
getFlip()
getGL()
Return the GL instance allowing to invoke OpenGL.Return the event listener handling init, drawing and resize requests.Return the performance information about this panel.Image<?>
boolean
Return true if the offscreen renderer has been initialized, which means that this panel has been added to a parent component.boolean
Return true if display has started but has not yet finishedprotected void
Show performance in a 2D text overlay.void
Invoked only for redraw query that are not coalesced with other redraw queries by the AWT Event Queue.void
Render GL image and stop counting elapsed time for rendering (started atdisplay()
)void
Called before the JPanel is removed from the Swing hierarchy.void
void
setFlip
(GLCanvas.Flip flip) void
setGLEventListener
(GLEventListener glEvents) Set the event listener handling init, drawing and resize requests.void
setMonitoring
(RenderCounter counter) void
setOffscreenRenderer
(OffscreenRenderer offscreen) protected void
setRendering
(boolean status) void
setScreenshot
(Image<?> image) void
Invoked each time redraw should be performed, even if the redraw query is coalesced with other redraw queries by the AWT Event Queue.Methods inherited from class javax.swing.JPanel
getAccessibleContext, getUI, getUIClassID, paramString, setUI, updateUI
Methods inherited from class javax.swing.JComponent
addAncestorListener, addVetoableChangeListener, computeVisibleRect, contains, createToolTip, disable, enable, firePropertyChange, firePropertyChange, firePropertyChange, fireVetoableChange, getActionForKeyStroke, getActionMap, getAlignmentX, getAlignmentY, getAncestorListeners, getAutoscrolls, getBaseline, getBaselineResizeBehavior, getBorder, getBounds, getClientProperty, getComponentGraphics, getComponentPopupMenu, getConditionForKeyStroke, getDebugGraphicsOptions, getDefaultLocale, getFontMetrics, getGraphics, getHeight, getInheritsPopupMenu, getInputMap, getInputMap, getInputVerifier, getInsets, getInsets, getListeners, getLocation, getMaximumSize, getMinimumSize, getNextFocusableComponent, getPopupLocation, getPreferredSize, getRegisteredKeyStrokes, getRootPane, getSize, getToolTipLocation, getToolTipText, getToolTipText, getTopLevelAncestor, getTransferHandler, getVerifyInputWhenFocusTarget, getVetoableChangeListeners, getVisibleRect, getWidth, getX, getY, grabFocus, hide, isDoubleBuffered, isLightweightComponent, isManagingFocus, isOpaque, isOptimizedDrawingEnabled, isPaintingForPrint, isPaintingOrigin, isPaintingTile, isRequestFocusEnabled, isValidateRoot, paintBorder, paintChildren, paintImmediately, paintImmediately, print, printAll, printBorder, printChildren, printComponent, processComponentKeyEvent, processKeyBinding, processKeyEvent, processMouseEvent, processMouseMotionEvent, putClientProperty, registerKeyboardAction, registerKeyboardAction, removeAncestorListener, removeVetoableChangeListener, repaint, repaint, requestDefaultFocus, requestFocus, requestFocus, requestFocusInWindow, requestFocusInWindow, resetKeyboardActions, reshape, revalidate, scrollRectToVisible, setActionMap, setAlignmentX, setAlignmentY, setAutoscrolls, setBackground, setBorder, setComponentPopupMenu, setDebugGraphicsOptions, setDefaultLocale, setDoubleBuffered, setEnabled, setFocusTraversalKeys, setFont, setForeground, setInheritsPopupMenu, setInputMap, setInputVerifier, setMaximumSize, setMinimumSize, setNextFocusableComponent, setOpaque, setPreferredSize, setRequestFocusEnabled, setToolTipText, setTransferHandler, setUI, setVerifyInputWhenFocusTarget, setVisible, unregisterKeyboardAction
Methods inherited from class java.awt.Container
add, add, add, add, add, addContainerListener, addImpl, addPropertyChangeListener, addPropertyChangeListener, applyComponentOrientation, areFocusTraversalKeysSet, countComponents, deliverEvent, doLayout, findComponentAt, findComponentAt, getComponent, getComponentAt, getComponentAt, getComponentCount, getComponents, getComponentZOrder, getContainerListeners, getFocusTraversalKeys, getFocusTraversalPolicy, getLayout, getMousePosition, insets, invalidate, isAncestorOf, isFocusCycleRoot, isFocusCycleRoot, isFocusTraversalPolicyProvider, isFocusTraversalPolicySet, layout, list, list, locate, minimumSize, paintComponents, preferredSize, printComponents, processContainerEvent, processEvent, remove, remove, removeAll, removeContainerListener, setComponentZOrder, setFocusCycleRoot, setFocusTraversalPolicy, setFocusTraversalPolicyProvider, setLayout, transferFocusDownCycle, validate, validateTree
Methods inherited from class java.awt.Component
action, add, addComponentListener, addFocusListener, addHierarchyBoundsListener, addHierarchyListener, addInputMethodListener, addKeyListener, addMouseListener, addMouseMotionListener, addMouseWheelListener, bounds, checkImage, checkImage, coalesceEvents, contains, createImage, createImage, createVolatileImage, createVolatileImage, disableEvents, dispatchEvent, enable, enableEvents, enableInputMethods, firePropertyChange, firePropertyChange, firePropertyChange, firePropertyChange, firePropertyChange, firePropertyChange, getBackground, getBounds, getColorModel, getComponentListeners, getComponentOrientation, getCursor, getDropTarget, getFocusCycleRootAncestor, getFocusListeners, getFocusTraversalKeysEnabled, getFont, getForeground, getGraphicsConfiguration, getHierarchyBoundsListeners, getHierarchyListeners, getIgnoreRepaint, getInputContext, getInputMethodListeners, getInputMethodRequests, getKeyListeners, getLocale, getLocation, getLocationOnScreen, getMouseListeners, getMouseMotionListeners, getMousePosition, getMouseWheelListeners, getName, getParent, getPropertyChangeListeners, getPropertyChangeListeners, getSize, getToolkit, getTreeLock, gotFocus, handleEvent, hasFocus, imageUpdate, inside, isBackgroundSet, isCursorSet, isDisplayable, isEnabled, isFocusable, isFocusOwner, isFocusTraversable, isFontSet, isForegroundSet, isLightweight, isMaximumSizeSet, isMinimumSizeSet, isPreferredSizeSet, isShowing, isValid, isVisible, keyDown, keyUp, list, list, list, location, lostFocus, mouseDown, mouseDrag, mouseEnter, mouseExit, mouseMove, mouseUp, move, nextFocus, paintAll, postEvent, prepareImage, prepareImage, processComponentEvent, processFocusEvent, processHierarchyBoundsEvent, processHierarchyEvent, processInputMethodEvent, processMouseWheelEvent, remove, removeComponentListener, removeFocusListener, removeHierarchyBoundsListener, removeHierarchyListener, removeInputMethodListener, removeKeyListener, removeMouseListener, removeMouseMotionListener, removeMouseWheelListener, removePropertyChangeListener, removePropertyChangeListener, repaint, repaint, repaint, requestFocus, requestFocus, requestFocusInWindow, resize, resize, setBounds, setBounds, setComponentOrientation, setCursor, setDropTarget, setFocusable, setFocusTraversalKeysEnabled, setIgnoreRepaint, setLocale, setLocation, setLocation, setMixingCutoutShape, setName, setSize, setSize, show, show, size, toString, transferFocus, transferFocusBackward, transferFocusUpCycle
-
Field Details
-
listener
-
offscreen
-
out
-
rendering
-
counter
-
overlay
-
debug
protected boolean debug -
debugPerf
protected boolean debugPerf -
flip
-
-
Constructor Details
-
GLCanvasSwing
public GLCanvasSwing() -
GLCanvasSwing
Initialize a panel able to render OpenGL through aGLEventListener
and relatedGL
interface.
-
-
Method Details
-
addNotify
public void addNotify()Called after the JPanel has been added to the Swing hierarchy but before it is made visible. Initialization may occur in other threads and not be completed when this method returns. To ensure the component is initialized, callinvalid @link
invalid input: ''
- Overrides:
addNotify
in classJComponent
-
removeNotify
public void removeNotify()Called before the JPanel is removed from the Swing hierarchy.- Overrides:
removeNotify
in classJComponent
-
update
Invoked each time redraw should be performed, even if the redraw query is coalesced with other redraw queries by the AWT Event Queue.- Overrides:
update
in classJComponent
-
paint
Invoked only for redraw query that are not coalesced with other redraw queries by the AWT Event Queue.- Overrides:
paint
in classJComponent
-
paintComponent
Render GL image and stop counting elapsed time for rendering (started atdisplay()
)- Overrides:
paintComponent
in classJComponent
-
display
public void display()If the panel initialization has achieved, this triggers an offscreen rendering, maybe on a separated thread (macOS case), from which an asynchronous repaint will be triggered. -
isInitialized
public boolean isInitialized()Return true if the offscreen renderer has been initialized, which means that this panel has been added to a parent component.- Specified by:
isInitialized
in interfaceGLCanvas
-
isRendering
public boolean isRendering()Return true if display has started but has not yet finished- Specified by:
isRendering
in interfaceGLCanvas
-
setRendering
protected void setRendering(boolean status) -
overlayPerformance
Show performance in a 2D text overlay. -
getGLEventListener
Description copied from interface:GLCanvas
Return the event listener handling init, drawing and resize requests.- Specified by:
getGLEventListener
in interfaceGLCanvas
-
setGLEventListener
Description copied from interface:GLCanvas
Set the event listener handling init, drawing and resize requests.- Specified by:
setGLEventListener
in interfaceGLCanvas
-
getGL
Description copied from interface:GLCanvas
Return the GL instance allowing to invoke OpenGL. -
getContext
Description copied from interface:GLCanvas
Return the GL context.- Specified by:
getContext
in interfaceGLCanvas
-
getScreenshot
- Specified by:
getScreenshot
in interfaceGLCanvas
-
setScreenshot
- Specified by:
setScreenshot
in interfaceGLCanvas
-
getFBO
-
setFBO
-
getMonitoring
Description copied from interface:GLCanvas
Return the performance information about this panel.- Specified by:
getMonitoring
in interfaceGLCanvas
-
setMonitoring
-
getOffscreenRenderer
- Specified by:
getOffscreenRenderer
in interfaceGLCanvas
-
setOffscreenRenderer
- Specified by:
setOffscreenRenderer
in interfaceGLCanvas
-
getFlip
-
setFlip
-